Table of Contents
Keywords in JavaScript play a crucial role in enabling developers to work with functions and objects effectively. Among these keywords, “call,” “apply,” and “bind” are widely used and offer powerful functionality for manipulating functions and their contexts. In this article, we will explore the purpose and usage of these keyword methods and how they can enhance your JavaScript coding skills.
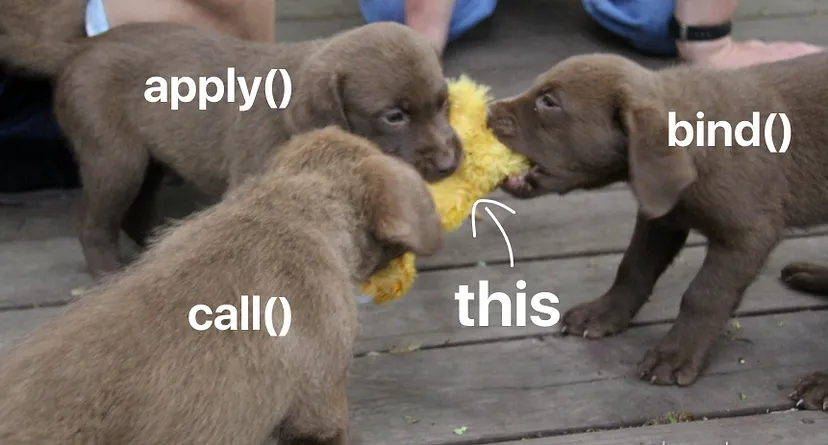
When working with JavaScript functions, you may come across situations where you need to change the context of a function or explicitly set the value of this
inside a function. This is where the “call,” “apply,” and “bind” methods come into play. They provide a way to manipulate function execution and establish the desired context.
Understanding Function Context
Before diving into the specifics of each keyword method, it’s essential to understand the concept of function context in JavaScript. In JavaScript, every function has a context, often represented by the this
keyword. The value of this
inside a function depends on how the function is called or invoked. By default, this
refers to the global object (e.g., window
in a browser or global
in Node.js).
The “Call” Method
The “call” method is used to invoke a function explicitly, allowing you to pass a specific object as the context for the function execution. The syntax for the “call” method is as follows:
functionName.call(context, arg1, arg2, ...);
Here, context
represents the object that will be used as the context for the function execution. Additional arguments can be passed to the function as well.
The “Apply” Method
Similar to the “call” method, the “apply” method is used to invoke a function explicitly. However, instead of passing individual arguments, it accepts an array-like object as the second argument, which will be used as the function’s arguments. The syntax for the “apply” method is as follows:
functionName.apply(context, [arg1, arg2, ...]);
The “apply” method is particularly useful when the number of arguments is unknown or variable.
The “Bind” Method
The “bind” method is used to create a new function that is bound to a specific context. Unlike the “call” and “apply” methods, which immediately invoke the function, the “bind” method returns a new function with the specified context. The syntax for the “bind” method is as follows:
var newFunction = functionName.bind(context);
The newly created newFunction
will always have context
as its this
value when invoked.
Differences Between “Call,” “Apply,” and “Bind”
Although “call,” “apply,” and “bind” serve similar purposes, they differ in their invocation and return values. The “call” and “apply” methods directly invoke the function, while the “bind” method returns a new function without executing it immediately. Furthermore, “call” and “apply” expect individual arguments or an array-like object, respectively, while “bind” only accepts the context.
Use Cases and Examples
The keyword methods “call,” “apply,” and “bind” have numerous practical use cases in JavaScript development. Here are a few examples:
1. Modifying the Context:
You can change the context of a function by using “call” or “apply,” allowing you to use a method from one object on another object.
Example:
const person1 = {
name: 'John',
sayHello: function () {
console.log(`Hello, ${this.name}!`);
}
};
const person2 = {
name: 'Jane'
};
person1.sayHello(); // Output: Hello, John!
person1.sayHello.call(person2); // Output: Hello, Jane!
In this example, we have two objects, person1
and person2
. The sayHello
method is defined on person1
and logs a greeting using the name
property. By using the “call” method, we invoke the sayHello
method with person2
as the context, resulting in a different greeting.
2. Borrowing Methods:
With “call” or “apply,” you can borrow methods from one object and apply them to another object.
Example:
const calculator = {
calculate: function (a, b) {
return a + b;
}
};
const numbers = [3, 5];
const result = calculator.calculate.apply(null, numbers);
console.log(result); // Output: 8
In this example, we have a calculator
object with a calculate
method that performs addition. Instead of passing individual arguments to the calculate
method, we use the “apply” method to pass the elements of the numbers
array as arguments. By passing null
as the context, we ensure that the method is not dependent on any specific object.
3. Function Currying:
The “bind” method allows you to create a partially applied function by fixing some arguments, which is useful in functional programming.
Example:
function greet(greeting, name) {
console.log(`${greeting}, ${name}!`);
}
const greetHello = greet.bind(null, 'Hello');
greetHello('John'); // Output: Hello, John!
const greetHi = greet.bind(null, 'Hi');
greetHi('Jane'); // Output: Hi, Jane!
In this example, we have a greet
function that takes a greeting
and name
as arguments and logs a personalized greeting. By using the “bind” method, we create new functions, greetHello
and greetHi
, that have the greeting
argument partially applied. This technique, known as function currying, allows us to create reusable functions with fixed arguments.
These examples demonstrate different scenarios where the “call,” “apply,” and “bind” methods can be utilized to modify function context, borrow methods, or create partially applied functions. Experimenting with these methods will provide you with a deeper understanding of their capabilities and how they can optimize your JavaScript code.
Best Practices for Using “Call,” “Apply,” and “Bind
To ensure the proper usage of “call,” “apply,” and “bind” in your code, consider the following best practices:
- Understand the Context: Familiarize yourself with the concept of function context and determine when it is necessary to manipulate it using these methods.
- Use Properly Named Variables: Choose meaningful names for the context and arguments to improve code readability.
- Be Mindful of Performance: Repeatedly invoking “bind” can lead to unnecessary function creations, impacting performance. Consider using it sparingly or finding alternative approaches.
Potential Caveats and Considerations
While “call,” “apply,” and “bind” are powerful tools, it’s crucial to keep a few considerations in mind:
- Overusing Function Context Manipulation: Excessive reliance on these methods can make your code harder to understand and maintain. Use them judiciously and consider alternative approaches when appropriate.
- Compatibility: “Call,” “apply,” and “bind” are available in modern JavaScript environments. However, if you need to support older browsers, ensure compatibility or use polyfills.
Conclusion
In conclusion, the keyword methods “call,” “apply,” and “bind” are valuable additions to your JavaScript toolkit. They provide flexibility in function execution and allow you to control the context in which functions are invoked. By understanding their usage and best practices, you can write more efficient and concise code.
FAQs
Can I use “call” and “apply” interchangeably?
No, “call” and “apply” differ in the way they accept function arguments. “Call” expects individual arguments, while “apply” accepts an array-like object.
How is “bind” different from “call” and “apply”?
“Bind” returns a new function with the specified context but does not immediately invoke it. “Call” and “apply” directly invoke the function with the provided context.
Can I use “call,” “apply,” and “bind” with arrow functions?
No, arrow functions in JavaScript do not have their own this
value and do not support the “call,” “apply,” or “bind” methods.
Are “call,” “apply,” and “bind” only used with objects?
No, while these methods are commonly used with objects, you can also use them with functions, allowing you to control the function context dynamically.
How do “call,” “apply,” and “bind” affect function performance?
Using these methods introduces a slight performance overhead compared to a regular function invocation. However, the impact is negligible in most cases, and the benefits they provide often outweigh the minimal performance difference.
Add your first comment to this post