Table of Contents
In the world of web development, JavaScript is a powerful programming language that enhances the functionality and interactivity of websites. However, due to its flexible nature, JavaScript can sometimes lead to unexpected behaviors and hard-to-detect bugs. This is where JavaScript strict mode comes into play. In this article, we will explore what strict mode is, its benefits, and how to use it effectively to write clean and reliable code.
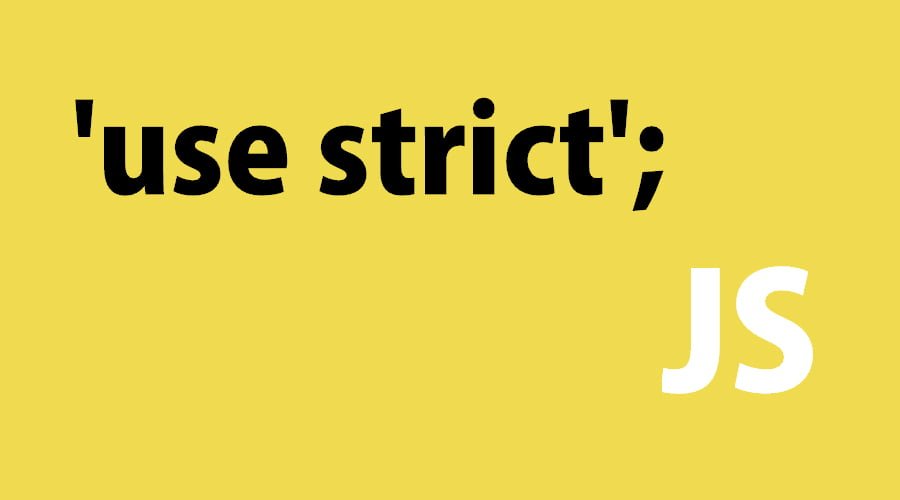
What is JavaScript strict mode?
JavaScript strict mode is a feature introduced in ECMAScript 5 (ES5) that allows developers to opt into a restricted variant of JavaScript. When enabled, strict mode enforces stricter rules for code execution, catching common mistakes and preventing potentially problematic behavior. It helps developers write cleaner and more reliable code by flagging and eliminating certain error-prone language features.
Enabling strict mode
Enabling strict mode is straightforward. You can activate it either globally for an entire script or locally within a specific function. To enable strict mode for an entire script, add the following directive at the beginning of your JavaScript file:
"use strict";
For local activation within a function, place the directive at the beginning of the function’s body. The strict mode directive applies to the function and its inner scope.
Benefits of using strict mode
1. Catching common coding mistakes
Strict mode helps catch common coding mistakes that would otherwise go unnoticed. It disallows the use of undeclared variables, prevents assignments to non-writable global variables, and throws errors on ambiguous syntax.
2. Enhancing security
By eliminating potentially unsafe actions and deprecated features, strict mode enhances the security of your JavaScript code. It reduces the risk of vulnerabilities, such as accidentally exposing global variables or using deprecated functions that might have security issues.
3. Improving performance
Strict mode allows JavaScript engines to perform certain optimizations that can lead to improved runtime performance. With strict mode enabled, the engine can make assumptions about your code, resulting in faster execution and more efficient memory usage.
Restrictions and changes in strict mode
Strict mode introduces a set of restrictions and changes to the JavaScript language. These modifications help eliminate confusing or error-prone features. Let’s explore some of the notable differences when operating in strict mode.
1. Variables and assignments
In strict mode, you must explicitly declare variables using the var
, let
, or const
keywords. Assignments to undeclared variables and attempts to assign values to read-only properties will throw errors.
In non-strict mode:
x = 10; // Assigns 10 to a global variable 'x' (implicitly declared)
console.log(x); // Output: 10
In strict mode:
"use strict";
x = 10; // Throws an error: Uncaught ReferenceError: x is not defined
2. Functions and parameters
Strict mode applies stricter rules to function declarations and parameters. It disallows the use of duplicate parameter names, prevents the accidental global binding of function declarations, and treats the this
value as undefined
in functions declared without an explicit object reference.
In non-strict mode:
function greet(name) {
console.log("Hello, " + name);
}
greet("John"); // Output: Hello, John
greet("John", "Doe"); // Output: Hello, John (extra argument is ignored)
In strict mode:
"use strict";
function greet(name) {
console.log("Hello, " + name);
}
greet("John"); // Output: Hello, John
greet("John", "Doe"); // Throws an error: Uncaught TypeError: 2 arguments were passed, but only 1 expected
3. Octal literals
In non-strict mode, JavaScript interprets numbers with leading zeroes as octal literals. However, this behavior can lead to confusion and unintended consequences. Strict mode removes support for octal literals, throwing errors when encountered.
In non-strict mode:
var num = 0755; // Octal literal (493 in decimal)
console.log(num); // Output: 493
In strict mode:
"use strict";
var num = 0755; // Throws an error: Uncaught SyntaxError: Octal literals are not allowed in strict mode
4. Deprecated features
Strict mode disables certain deprecated features, such as the use of the with
statement, which can cause scope-related issues and potential security risks. It also restricts the use of the arguments.callee
property and prevents redefining functions.
In non-strict mode:
var obj = { value: 10 };
with (obj) {
console.log(value); // Output: 10
}
In strict mode:
"use strict";
var obj = { value: 10 };
with (obj) {
console.log(value); // Throws an error: Uncaught SyntaxError: Strict mode code may not include a with statement
}
These examples demonstrate some of the differences and restrictions introduced by JavaScript strict mode. By adhering to strict mode, you can ensure cleaner and more reliable code that follows modern best practices.
Migrating existing code to strict mode
When adopting strict mode, it’s essential to consider existing codebases. Migrating older code to strict mode requires careful analysis and potential modifications to comply with the stricter rules. Here are some steps to follow when migrating existing code to strict mode:
1. Identifying potential issues
Start by identifying potential issues that strict mode might expose. These can include undeclared variables, ambiguous syntax, or deprecated language features. Analyze your codebase thoroughly and make a list of areas that may need attention during the migration process.
2. Updating code to comply with strict mode
Once potential issues are identified, update the code to comply with strict mode. This may involve declaring variables explicitly, addressing syntax ambiguities, and replacing deprecated features with modern alternatives. Testing and verifying the code after modification is crucial to ensure its continued functionality.
Common misconceptions about strict mode
There are a few misconceptions surrounding strict mode that are worth debunking. Let’s address some of the common misunderstandings:
- Misconception 1: Strict mode makes JavaScript faster. While strict mode enables some performance optimizations, the difference in speed is usually negligible in real-world scenarios.
- Misconception 2: Strict mode is only useful for catching errors. While error detection is an essential aspect of strict mode, its benefits go beyond that. It promotes good coding practices, enhances security, and improves code readability.
- Misconception 3: Strict mode is a new version of JavaScript. Strict mode is a feature within JavaScript and not a separate version. It helps enforce a stricter subset of the language to prevent potential issues.
Best practices for using strict mode
To get the most out of strict mode, consider the following best practices:
1. Always enable strict mode
Make it a habit to enable strict mode for all your JavaScript files. This ensures consistent code behavior and helps catch potential errors early in the development process.
2. Use strict mode for all new code
When writing new code, start in strict mode right from the beginning. By defaulting to strict mode, you establish a clean and reliable foundation for your project.
3. Understand the impact on third-party libraries
If your project relies on third-party libraries or frameworks, ensure they are compatible with strict mode. Some libraries might not be strict-mode-ready, so it’s crucial to test and verify their behavior in strict mode before integrating them into your project.
3. Regularly review and update code
As your codebase evolves, periodically review and update it to maintain strict mode compliance. This includes addressing any warnings or errors flagged by modern JavaScript development tools and keeping up with the latest best practices.
Conclusion
JavaScript strict mode is a valuable tool that allows developers to write cleaner, more reliable code. By enabling strict mode, you can catch common coding mistakes, enhance the security of your applications, and improve performance. It’s crucial to understand the restrictions and changes introduced by strict mode and adopt best practices for its effective usage.
FAQs
What happens if I don’t enable strict mode in JavaScript?
Without enabling strict mode, JavaScript operates in non-strict mode, where certain errors can go unnoticed, and potentially problematic language features are allowed. Enabling strict mode helps catch these errors and promotes better coding practices.
Can I use strict mode in specific parts of my code?
Yes, you can enable strict mode globally for an entire script or locally within specific functions. By placing the strict mode directive appropriately, you can control the scope in which strict mode applies.
Does strict mode make JavaScript faster?
While strict mode enables some performance optimizations, the difference in speed is usually negligible in typical scenarios. The primary benefit of strict mode lies in catching errors and promoting code reliability.
Can I use strict mode with older versions of JavaScript?
Strict mode was introduced in ECMAScript 5 (ES5). If you’re using an older version of JavaScript, strict mode may not be available. It’s recommended to use the latest version of JavaScript to leverage strict mode and other modern language features.
Where can I learn more about strict mode and JavaScript best practices?
There are various online resources, tutorials, and documentation available to learn more about strict mode and JavaScript best practices. Additionally, community forums and developer communities can provide valuable insights and guidance.
Add your first comment to this post