Table of Contents
Arrays are an essential part of programming languages, providing a way to store and manipulate collections of data. In JavaScript, arrays come with a variety of built-in methods that offer powerful functionalities. This article explores six important array methods: slice, splice, reverse, concat, join, and at. By understanding how these methods work, you can enhance your programming skills and improve your code efficiency.
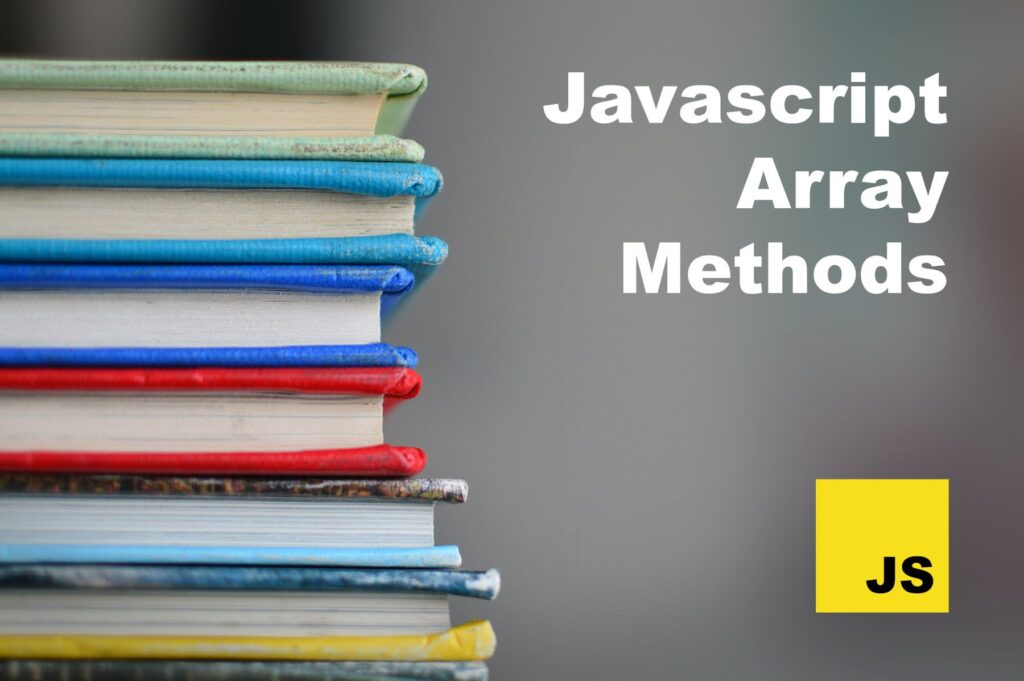
Slice
The slice
method in JavaScript allows you to extract a portion of an array without modifying the original array. It takes two optional parameters: the starting index and the ending index. By specifying the appropriate indices, you can extract a subarray containing the desired elements.
const fruits = ['apple', 'banana', 'orange', 'kiwi', 'mango'];
const citrusFruits = fruits.slice(2, 4);
console.log(citrusFruits); // Output: ['orange', 'kiwi']
Splice
The splice
method is used to add or remove elements from an array. It can be used to remove elements by specifying the starting index and the number of elements to remove. Additionally, you can also add elements by providing the index, the number of elements to remove, and the elements to add.
Example of removing elements:
const numbers = [1, 2, 3, 4, 5];
numbers.splice(2, 2);
console.log(numbers); // Output: [1, 2, 5]
Example of adding elements:
const colors = ['red', 'blue', 'green'];
colors.splice(1, 0, 'yellow', 'orange');
console.log(colors); // Output: ['red', 'yellow', 'orange', 'blue', 'green']
Reverse
The reverse
method reverses the order of elements in an array. It modifies the original array, so use it with caution if you want to preserve the original order.
Example:
const numbers = [1, 2, 3, 4, 5];
numbers.reverse();
console.log(numbers); // Output: [5, 4, 3, 2, 1]
Concat
The concat
method allows you to merge two or more arrays into a single array. It does not modify the original arrays; instead, it returns a new array containing the concatenated elements.
Example:
const arr1 = ['a', 'b', 'c'];
const arr2 = ['d', 'e', 'f'];
const mergedArray = arr1.concat(arr2);
console.log(mergedArray); // Output: ['a', 'b', 'c', 'd', 'e', 'f']
Join
The join
method is used to create a string from the elements of an array. It concatenates the elements using a specified separator and returns the resulting string.
Example:
const fruits = ['apple', 'banana', 'orange'];
const fruitsString = fruits.join(', ');
console.log(fruitsString); // Output: 'apple, banana, orange'
At
The at
method is not a built-in array method in JavaScript, but it can be implemented using array indexing. It allows you to access an element at a specific index in an array.
Example:
const numbers = [1, 2, 3, 4, 5];
const element = numbers[2];
console.log(element); // Output: 3
Conclusion
Understanding array methods is crucial for efficient array manipulation in JavaScript. In this article, we explored six important array methods: slice, splice, reverse, concat, join, and at. By leveraging these methods, you can perform a wide range of operations on arrays, from extracting subarrays to adding or removing elements. Remember to choose the appropriate method based on your specific requirements to optimize your code.
FAQs
Can I use the slice
method to extract elements from an array without specifying the ending index?
Yes, if you omit the ending index, the slice
method will extract all elements from the starting index to the end of the array.
Does the splice
method modify the original array?
Yes, the splice
method modifies the original array by adding or removing elements.
Is it possible to reverse the order of elements in an array without modifying the original array?
No, the reverse
method modifies the original array in place.
Can I concatenate more than two arrays using the concat
method?
Yes, you can concatenate multiple arrays by providing them as arguments to the concat
method.
How can I access an element at a specific index in an array?
You can use array indexing, such as array[index]
, to access an element at a particular index in an array.
Add your first comment to this post