Table of Contents
In the realm of JavaScript programming, developers often come across various coding techniques and patterns that enhance code organization, encapsulation, and maintainability. One such technique is the Immediately Invoked Function Expression (IIFE). In this article, we will delve into the concept of IIFE, its benefits, and provide coding examples to illustrate its usage.
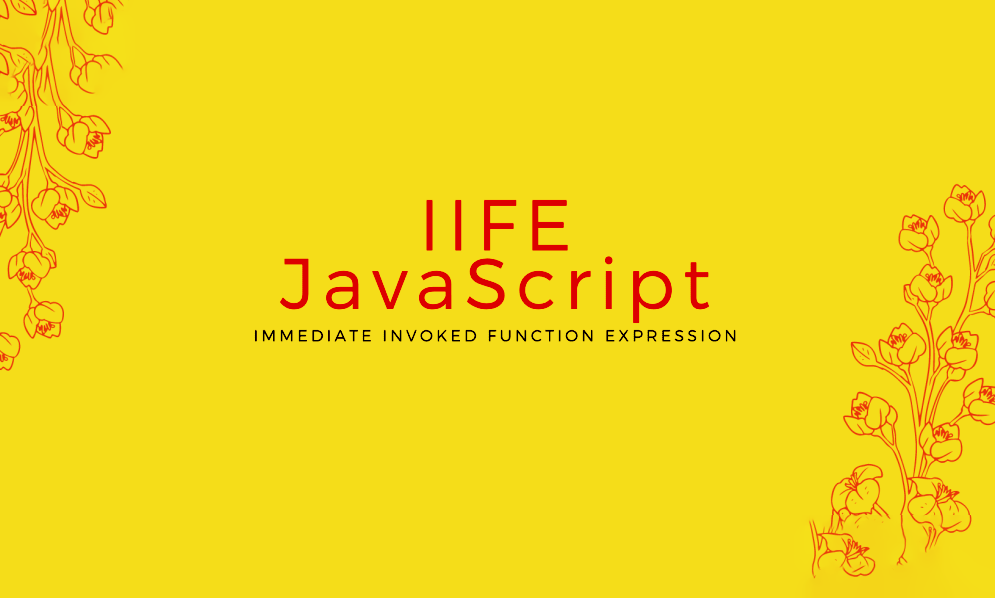
The Immediately Invoked Function Expression (IIFE) is a JavaScript function that is executed immediately after it is defined. It is a self-invoking anonymous function that allows you to encapsulate code and avoid polluting the global scope. By wrapping your code within an IIFE, you create a private scope where variables and functions can be declared without affecting the global namespace.
Syntax of IIFE
To create an IIFE, you can use the following syntax:
(function(){
// Your code here
})();
The outer parentheses around the function declaration turn it into an expression, and the trailing parentheses invoke the function immediately.
Here’s an example of an IIFE that prints a message to the console:
(function(){
var message = "Hello, IIFE!";
console.log(message);
})();
The Purpose and Advantages of IIFE
2. Encapsulation and Avoiding Global Scope Pollution
One of the primary purposes of using IIFE is to encapsulate code and prevent variable and function name clashes in the global scope. By wrapping your code in an IIFE, you create a private scope where your variables and functions are confined, reducing the chances of unintended conflicts with other code.
Here’s an example that demonstrates encapsulation using IIFE:
(function(){
var count = 0;
function increment() {
count++;
console.log("Count:", count);
}
increment();
})();
2. Data Privacy
Another advantage of using IIFE is that it allows you to create private variables and functions that are not accessible from outside the IIFE. This concept of data privacy is crucial in maintaining the integrity and security of your code.
Here’s an example that showcases data privacy with IIFE:
var calculator = (function(){
var total = 0;
function add(number) {
total += number;
}
function subtract(number) {
total -= number;
}
function getTotal() {
return total;
}
return {
add: add,
subtract: subtract,
getTotal: getTotal
};
})();
calculator.add(5);
calculator.subtract(2);
console.log("Total:", calculator.getTotal());
IIFE Use Cases
IIFE finds its application in various scenarios, including:
1. Creating Closures
By utilizing IIFE, you can create closures to maintain the state of variables. This is particularly useful when dealing with asynchronous operations or when you want to preserve the value of a variable for future use.
Here’s an example that demonstrates the use of IIFE to create a closure:
var button = document.querySelector("button");
(function(){
var count = 0;
button.addEventListener("click", function(){
count++;
console.log("Button clicked", count, "times");
});
})();
2. Reducing Global Scope Pollution
When working on large-scale JavaScript projects, minimizing global scope pollution becomes crucial. IIFE provides an effective solution by encapsulating code and reducing the likelihood of naming conflicts.
Here’s an example that showcases the reduction of global scope pollution with IIFE:
(function(){
// Code for a specific module
// Variables and functions specific to this module
var moduleVariable = "Module";
function moduleFunction() {
console.log("Module function");
}
// Rest of the module code
})();
3. Module Pattern Implementation
IIFE is widely used in implementing the Module pattern in JavaScript. The Module pattern allows you to create self-contained modules with private variables and functions, exposing only the necessary public API.
Here’s an example of implementing the Module pattern using IIFE:
var module = (function(){
var privateVariable = "Private";
function privateFunction() {
console.log("Private function");
}
function publicFunction() {
console.log("Public function");
}
return {
publicFunction: publicFunction
};
})();
module.publicFunction();
Common Mistakes to Avoid with IIFE
While working with IIFE, it’s essential to keep a few things in mind to avoid common mistakes:
1. Missing Parentheses
Ensure that you wrap the IIFE in parentheses to make it an expression and invoke it immediately. Forgetting the parentheses can result in syntax errors or unexpected behavior.
2. Not Passing Arguments Properly
If your IIFE requires any arguments, make sure to pass them correctly when invoking the function. Failing to do so can lead to unexpected results or errors within your code.
3. Neglecting the Return Value
Remember to handle the return value of an IIFE if you expect it to produce a result. Neglecting the return value can lead to issues when utilizing the IIFE’s output.
Conclusion
In conclusion, the Immediately Invoked Function Expression (IIFE) is a powerful tool in JavaScript that allows you to encapsulate code, avoid global scope pollution, and create private variables and functions. By utilizing IIFE, you can improve code organization, enhance security, facilitate modular development, and create closures. Understanding the syntax, purpose, and advantages of IIFE is essential for every JavaScript developer striving to write clean, maintainable, and efficient code.
Frequently Asked Questions
What is the difference between IIFE and a regular function?
A regular function in JavaScript is defined with a name and is invoked when called explicitly. On the other hand, an IIFE is an anonymous function that is immediately invoked without the need for explicit invocation.
Can I pass arguments to an IIFE?
Yes, you can pass arguments to an IIFE. Simply provide the arguments within the parentheses at the end of the function declaration.
Can I access variables defined within an IIFE from outside?
No, variables defined within an IIFE are not accessible from outside the function. They are confined to the scope of the IIFE, providing data privacy.
Is using IIFE still relevant with the introduction of block-scoped variables in ES6?
Although block-scoped variables in ES6 provide an alternative to some use cases of IIFE, IIFE remains relevant for encapsulating code, maintaining backward compatibility, and reducing global scope pollution.
Can I nest multiple IIFEs within each other?
Yes, you can nest multiple IIFEs within each other to create a hierarchy of private scopes. This technique is often used in more complex code scenarios.
Add your first comment to this post