Table of Contents
JavaScript is a versatile programming language that is widely used for web development. One of the fundamental data types in JavaScript is the string. In this article, we will explore JavaScript strings in detail and understand their properties, manipulation techniques, and common use cases. So, let’s dive into the world of JavaScript strings!
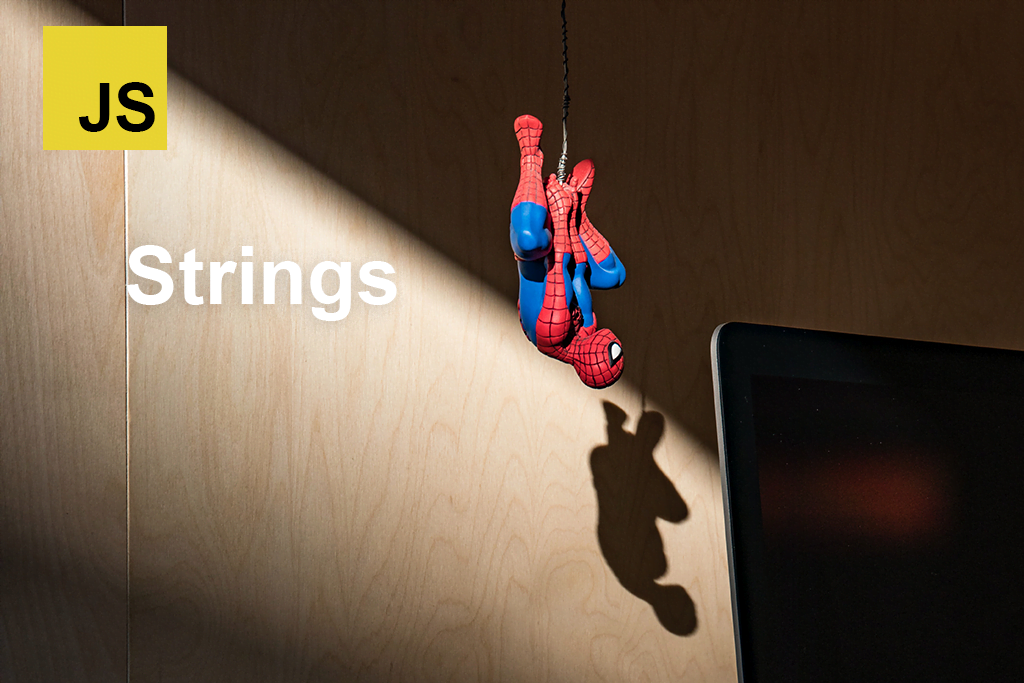
In JavaScript, a string is a sequence of characters enclosed within single quotes (”) or double quotes (“”). Strings can contain any combination of letters, numbers, symbols, or even special characters. They are immutable, meaning once a string is created, it cannot be changed. However, you can perform various operations on strings to extract information or modify their contents.
Creating and initializing strings
To create a string in JavaScript, you can simply assign a value to a variable using quotes. Here’s an example:
let message = "Hello, world!";
You can also create an empty string and append characters to it later:
let emptyString = "";
emptyString += "This is ";
emptyString += "an example.";
String properties and methods
JavaScript provides several properties and methods that allow you to work with strings effectively. Some commonly used properties include length
, which returns the number of characters in a string, and toUpperCase()
and toLowerCase()
, which convert the string to uppercase or lowercase, respectively.
let str = "JavaScript";
console.log(str.length); // Outputs 10
console.log(str.toUpperCase()); // Outputs "JAVASCRIPT"
console.log(str.toLowerCase()); // Outputs "javascript"
Concatenating strings
Concatenation is the process of combining two or more strings. In JavaScript, you can use the +
operator or the concat()
method to concatenate strings. Here’s an example:
let firstName = "John";
let lastName = "Doe";
let fullName = firstName + " " + lastName; // Using the + operator
let greeting = firstName.concat(" ", lastName); // Using the concat() method
Extracting substrings
To extract a portion of a string, you can use the substring()
or slice()
methods. These methods allow you to specify the start and end positions of the substring you want to extract. Here’s an example:
let str = "JavaScript is awesome";
let substring1 = str.substring(0, 10); // Extracts "JavaScript"
let substring2 = str.slice(11); // Extracts "is awesome"
Modifying strings
While strings are immutable, you can still modify them indirectly by using string manipulation methods. For example, you can replace a specific substring within a string using the replace()
method:
let str = "Hello, world!";
let newStr = str.replace("world", "JavaScript"); // Replaces "world" with "JavaScript"
Searching within strings
JavaScript provides various methods to search for specific patterns or characters within a string. The indexOf()
method returns the index of the first occurrence of a specified value, while the lastIndexOf()
method returns the index of the last occurrence. Here’s an example:
let str = "Hello, world!";
console.log(str.indexOf("o")); // Outputs 4
console.log(str.lastIndexOf("o")); // Outputs 8
String comparison
When comparing strings in JavaScript, you need to be cautious because JavaScript performs string comparisons based on the Unicode values of the characters. The localeCompare()
method is commonly used for string comparison. It returns a negative value if the first string comes before the second, a positive value if it comes after, and 0 if they are equal.
let str1 = "apple";
let str2 = "banana";
console.log(str1.localeCompare(str2)); // Outputs a negative value (-1)
Formatting strings
JavaScript provides the toLocaleString()
method, which allows you to format numbers, dates, and currencies based on the user’s locale. This is particularly useful when building internationalized web applications.
let number = 123456.789;
console.log(number.toLocaleString()); // Outputs "123,456.789"
String interpolation
String interpolation is a convenient way to embed expressions within a string. In JavaScript, you can use template literals (enclosed in backticks) to achieve string interpolation. Here’s an example:
let name = "John";
let greeting = `Hello, ${name}!`; // Outputs "Hello, John!"
Unicode and JavaScript strings
JavaScript uses the Unicode character set, which allows it to handle characters from various writing systems. This means that JavaScript strings can represent characters from different languages, including non-Latin scripts and special characters.
Here are a few examples:
let japaneseString = "日本語";
let greekString = "Ελληνικά";
let arabicString = "العربية";
console.log(japaneseString.length); // Outputs 3
console.log(greekString.charAt(0)); // Outputs "Ε"
console.log(arabicString.charCodeAt(0)); // Outputs the Unicode value of the first character
Common string operations
JavaScript’s Unicode support allows developers to work with multilingual content and handle a wide range of characters seamlessly.
Splitting a string into an array of substrings:
let sentence = "This is a sentence.";
let words = sentence.split(" "); // Splits the sentence into an array of words
console.log(words); // Outputs ["This", "is", "a", "sentence."]
Trimming whitespace from the beginning and end of a string:
let paddedString = " Hello, world! ";
let trimmedString = paddedString.trim();
console.log(trimmedString); // Outputs "Hello, world!"
Extracting individual characters:
let word = "JavaScript";
console.log(word.charAt(2)); // Outputs "v"
console.log(word[4]); // Outputs "S"
JavaScript provides many more string operations, such as replacing substrings, counting occurrences, and checking if a string contains a specific pattern. These operations allow you to manipulate and extract information from strings efficiently.
Best practices for working with strings
When working with strings in JavaScript, it’s important to follow some best practices to ensure code readability and performance. Here are a few recommendations:
Use appropriate string manipulation methods: Instead of accessing characters directly using indices, use string methods like charAt()
, substring()
, or slice()
to manipulate and extract portions of strings. This improves code clarity and readability.
Avoid unnecessary string concatenation in loops: Concatenating strings inside a loop can be inefficient, as it creates new string objects repeatedly. Instead, consider using array joins or template literals to build the final string.
Handle user input and encoding properly: When accepting user input, ensure proper validation and encoding to prevent security vulnerabilities like cross-site scripting (XSS) attacks. Use encoding functions, such as encodeURIComponent()
, to safely include user input in URLs or HTML.
Consider localization and internationalization: If your application needs to support multiple languages or locales, consider using internationalization (i18n) libraries or techniques to handle translations, date formatting, and number formatting.
String performance considerations
While JavaScript provides powerful string manipulation capabilities, it’s important to be mindful of performance considerations, especially when dealing with large strings or frequent string operations. Here are a few performance tips:
Minimize string concatenation in loops: Concatenating strings inside loops can be slow, as it creates new string objects repeatedly. Instead, consider using an array to collect the substrings and join them at the end using Array.prototype.join()
.
Use array-based approaches for heavy string manipulation: If you need to perform extensive modifications on a string, consider converting it to an array and manipulating the array elements. This can be more efficient than repeatedly modifying the string directly.
Cache the length of the string: When iterating over a string, cache the length of the string outside the loop to avoid repeatedly calculating it in each iteration.
Consider using regular expressions efficiently: Regular expressions can be powerful for string matching and manipulation, but they can also be computationally expensive. Use them judiciously and optimize them where possible.
By following these best practices and considering performance implications, you can optimize your JavaScript code when working with strings, resulting in more efficient and maintainable applications.
Conclusion
JavaScript strings are a fundamental part of web development, enabling the manipulation and representation of textual data. In this article, we covered various aspects of JavaScript strings, including their creation, properties, manipulation techniques, and best practices. By understanding the power of JavaScript strings, you can enhance your ability to develop dynamic and interactive web applications.
FAQs
Can I use single quotes to create a string in JavaScript?
Yes, you can use either single quotes (”) or double quotes (“”) to create a string in JavaScript.
Are JavaScript strings mutable?
No, JavaScript strings are immutable, meaning they cannot be changed once created. However, you can perform operations on strings to extract or modify their contents.
How can I convert a string to uppercase in JavaScript?
You can use the toUpperCase()
method to convert a string to uppercase in JavaScript. For example, str.toUpperCase()
will return the uppercase version of the str
string.
What is string interpolation in JavaScript?
String interpolation allows you to embed expressions within a string. In JavaScript, you can use template literals (enclosed in backticks) for string interpolation.
What are some performance considerations when working with strings in JavaScript?
When working with strings, be mindful of frequent string concatenation operations and using regular expressions on large strings, as these can impact performance. Consider alternative approaches like arrays or string builders for improved performance.
Add your first comment to this post