Table of Contents
In the world of JavaScript programming, functions play a crucial role in writing efficient and reusable code. Two important concepts related to functions are higher-order functions and first-class functions. Although these terms might sound similar, they have distinct characteristics and purposes within the JavaScript programming language. In this article, we will explore the difference between higher-order functions and first-class functions in JavaScript, their features, and how they contribute to the flexibility and power of the language.
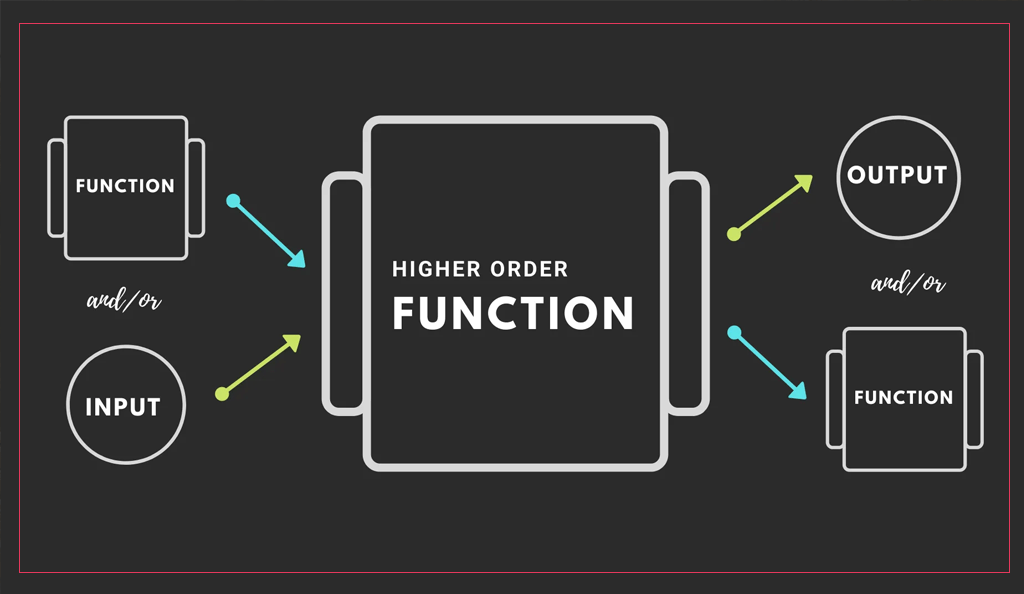
In the realm of JavaScript programming, functions are considered first-class citizens, and they form the building blocks of code organization and modularity. First-class functions and higher-order functions are concepts that take this notion a step further, providing JavaScript programmers with powerful tools to create flexible and expressive code.
What are First-Class Functions?
– Definition and Basic Features
First-class functions in JavaScript refer to the ability of the language to treat functions as values, enabling them to be assigned to variables, passed as arguments to other functions, and returned as results from functions. In simpler terms, functions are treated just like any other data type in the language.
– Storing Functions in Variables
One of the distinguishing features of first-class functions in JavaScript is the ability to store them in variables. This allows programmers to create references to functions and manipulate them as needed. For example:
const greet = function() {
console.log("Hello, world!");
}
const myFunction = greet;
myFunction(); // Outputs: Hello, world!
– Passing Functions as Arguments
Another significant characteristic of first-class functions is the ability to pass functions as arguments to other functions. This opens up possibilities for creating higher-order functions, which we will discuss shortly. Here’s an example:
function applyOperation(operation, a, b) {
return operation(a, b);
}
function sum(x, y) {
return x + y;
}
const result = applyOperation(sum, 3, 4); // Returns: 7
– Returning Functions from Functions
First-class functions in JavaScript also allow functions to return other functions as results. This is particularly useful when implementing complex algorithms or designing APIs with a high degree of flexibility. Here’s an example:
function createMultiplier(factor) {
return function(number) {
return number * factor;
}
}
const double = createMultiplier(2);
const result = double(5); // Returns: 10
What are Higher-Order Functions?
– Definition and Basic Features
Higher-order functions in JavaScript are functions that can accept other functions as parameters or return functions as results. In other words, they operate on functions, either by taking them as arguments or manipulating them in some way.
– Accepting Functions as Parameters
One of the key features of higher-order functions in JavaScript is their ability to accept other functions as parameters. This allows developers to define generic operations or behaviors that can be customized by providing different functions as arguments. Here’s an example:
function applyOperation(operation, a, b) {
return operation(a, b);
}
function multiply(x, y) {
return x * y;
}
const result = applyOperation(multiply, 3, 4); // Returns: 12
– Returning Functions as Results
Higher-order functions in JavaScript can also return functions as results. This enables the creation of factory functions or functions that generate specialized functions based on certain parameters. Here’s an example:
function createAdder(offset) {
return function(number) {
return number + offset;
}
}
const add5 = createAdder(5);
const result = add5(3); // Returns: 8
– Combining Functions
Higher-order functions in JavaScript can combine multiple functions to create new and more complex functionality. They can take functions as inputs, process them, and produce a new function as output. This allows for the composition of functions, leading to code that is modular, reusable, and easier to maintain.
Comparison between First-Class Functions and Higher-Order Functions
While first-class functions and higher-order functions in JavaScript are closely related, there are fundamental differences between them.
- First-class functions refer to the treatment of functions as values, allowing them to be assigned to variables, passed as arguments, and returned as results. It is a language-level characteristic in JavaScript.
- On the other hand, higher-order functions are functions that operate on other functions, either by accepting them as parameters or returning them as results. They are defined and implemented within the JavaScript language.
Despite these differences, first-class functions and higher-order functions share common characteristics:
- They both contribute to the expressiveness and flexibility of the JavaScript language.
- They enable the creation of more modular and reusable code.
- They empower JavaScript developers to implement advanced programming techniques, such as functional programming.
Advantages and Use Cases
– First-Class Functions
The ability to treat functions as first-class citizens in JavaScript offers several advantages, including:
- Function composition: Functions can be combined and composed to create more complex operations.
- Callbacks and event handling: Functions can be used as callbacks to handle events or asynchronous operations.
- Higher-level abstractions: Functions can be used to create higher-level abstractions and domain-specific languages.
Use cases for first-class functions in JavaScript include:
- Functional programming paradigms
- Event-driven programming
- Asynchronous programming
- Domain-specific languages (DSLs)
– Higher-Order Functions
Higher-order functions provide unique benefits in JavaScript, such as:
- Increased code modularity: Functions can be designed to operate on other functions, leading to more modular and reusable code.
- Customizable behavior: Higher-order functions allow developers to customize the behavior of certain operations or algorithms by providing different functions as arguments.
- Design patterns: Higher-order functions are often used to implement various design patterns, such as the Strategy pattern or the Decorator pattern.
Use cases for higher-order functions in JavaScript include:
- Implementing generic algorithms and operations
- Designing extensible frameworks or libraries
- Creating specialized functions based on different contexts
Conclusion
In summary, higher-order functions and first-class functions in JavaScript are powerful concepts that enhance code flexibility and reusability. First-class functions allow functions to be treated as values, while higher-order functions operate on functions themselves. Understanding the distinction between these concepts allows JavaScript developers to leverage their capabilities and create more expressive and efficient code.
Frequently Asked Questions (FAQs)
Are higher-order functions and first-class functions available in all programming languages?
Yes, the concepts of higher-order functions and first-class functions are supported in many modern programming languages, including JavaScript.
Can you provide an example of a use case where both higher-order functions and first-class functions are employed?
Certainly! One such example is in functional programming paradigms, where functions are treated as first-class citizens and higher-order functions are used to operate on those functions. This combination allows for elegant and concise code that emphasizes immutability and pure functions.
What is the advantage of using higher-order functions over regular functions?
Higher-order functions offer increased modularity and code reusability. They allow for the creation of generic algorithms that can work with different functions, providing a customizable and flexible approach to coding.
Can first-class functions and higher-order functions be used in object-oriented programming?
Absolutely! While these concepts are often associated with functional programming, they can be used alongside object-oriented programming paradigms to enhance code flexibility and modularity.
How do higher-order functions contribute to code maintainability?
By promoting code modularity and reusability, higher-order functions make code easier to understand, modify, and maintain. They encourage the separation of concerns and reduce code duplication.
Add your first comment to this post